scr_meta=<><![CDATA[ // ==UserScript== // @name Google Reader Sanity // @namespace cqrt // @description Restore your Google Reader interface to it's former glory and restore your sanity. -=Redesigned minimalist interface=- -=Inline Post Previews=- -=Reduced memory footprint=- -=Keyboard Shortcuts=- -=Collapsible Interface=- -=Auto Update=- -=True unread counts=- -=Enclosures restored=- // @icon https://www.google.com/reader/ui/352024653-app-icon-32.png // @icon64 https://www.google.com/reader/ui/3068170011-app-icon-64.png // @svc:version [1.9.0] // @version 1.9.0 // @history (1.9.0) True unread count, Inline post preview in 'List' mode, Tidy up 'List' view, Custom Logo, Beautified javascript // @updateURL http://userscripts.org/scripts/source/117298.meta.js // @include http://*.google.tld/reader/view/* // @include https://*.google.tld/reader/view/* // ==/UserScript== ]]></>.toString(); //document.getElementById("top-bar").className += " display-none"; document.getElementById("gb").className += " display-none"; document.getElementById("title-and-status-holder").className += " display-none"; //Fix Unread Count to show actual unread instead of 1000+ (function () { var isChrome = false; var isSafari = false; // features switch var hasDOMSubtreeModified = false; var unreadCountElement = null; function init() { if (navigator.userAgent.match(/Chrome/)) { isChrome = true; } else if (navigator.userAgent.match(/Safari/)) { isSafari = true; } hasDOMSubtreeModified = !isChrome && !isSafari; if (document.body) waitForReady(); } // Wait for the dom ready function waitForReady() { if (unreadCountElement = document.getElementById('reading-list-unread-count')) { if (hasDOMSubtreeModified) { var res = document.evaluate("//span[contains(@class, 'unread-count') and contains(@class, 'sub-unread-count') and not(contains(@class, 'folder-unread-count'))]", document, null, XPathResult.UNORDERED_NODE_SNAPSHOT_TYPE, null); for (var i = 0; i < res.snapshotLength; i++) { res.snapshotItem(i).parentNode.addEventListener('DOMSubtreeModified', modifySubtree, false); } window.addEventListener("DOMTitleChanged", calcUnread, false); } else { window.setInterval(calcUnread, 3000); } calcUnread(); } else { window.setTimeout(waitForReady, 500); } } function modifySubtree() { if (unreadCountElement.textContent.match(/\d{4}\+?/)) { calcUnread(); } } function findItemUnread(checkDuplicated, item) { var hasplus = false; var count = 0; var alreadyCounted = false; var countres = item.innerHTML.match(/\((\d*)\+?\)/); if (countres) { count = parseInt(countres[1], 10); if (item.innerHTML.match(/\(1000\+\)/)) { hasplus = true; } var nodeTitle = item.parentNode.getAttribute('title'); if (nodeTitle) { if (checkDuplicated.indexOf(nodeTitle) < 0) { checkDuplicated.push(nodeTitle); } else { alreadyCounted = true; } } } return { count: count, hasplus: hasplus, alreadyCounted: alreadyCounted }; } function calcUnread() { var checkDuplicated = []; var total = 0; var totalplus = false; var res = document.evaluate("//li[contains(@class, 'folder')]//li[contains(@class, 'folder')]", document, null, XPathResult.UNORDERED_NODE_SNAPSHOT_TYPE, null); for (var i = 0; i < res.snapshotLength; i++) { var res2 = document.evaluate(".//li[contains(@class, 'unread')]/a/div[contains(@class, 'unread-count')]", res.snapshotItem(i), null, XPathResult.UNORDERED_NODE_SNAPSHOT_TYPE, null); var subtotal = 0; var subtotalplus = false; for (var j = 0; j < res2.snapshotLength; j++) { var result = findItemUnread(checkDuplicated, res2.snapshotItem(j)); if (result.hasplus) { totalplus = true; subtotalplus = true; } subtotal += result.count; if (!result.alreadyCounted) { total += result.count; } } if (subtotal > 0) { var resfolder = document.evaluate(".//a/div[contains(@class, 'unread-count')]", res.snapshotItem(i), null, XPathResult.FIRST_ORDERED_NODE_TYPE, null).singleNodeValue; if (resfolder) { resfolder.innerHTML = ' (' + subtotal + (subtotalplus ? '+' : '') + ')'; } } } // untagged items var res2 = document.evaluate("//ul[@id='sub-tree']/li/ul/li[not(contains(@class, 'folder')) and contains(@class, 'unread')]/a/div[contains(@class, 'unread-count')]", document, null, XPathResult.UNORDERED_NODE_SNAPSHOT_TYPE, null); for (var j = 0; j < res2.snapshotLength; j++) { var result = findItemUnread(checkDuplicated, res2.snapshotItem(j)); if (result.hasplus) { totalplus = true; } if (!result.alreadyCounted) { total += result.count; } } if (total > 0) { var totaltext = total + (totalplus ? '+' : ''); unreadCountElement.innerHTML = ' (' + totaltext + ')'; // update windows title as well if (totaltext) { var newTitle = '(' + totaltext + ') ' + document.title.replace(/\s*\(\d+\+?\)$/, '').replace(/^\(\d+\+?\)\s*/, '');; if (document.title != newTitle) { document.title = newTitle; } } } } init(); })(); // Fix the image enclosure not displayed by Google Reader (function () { //object constructor function GoogleReaderFixer() { this.fixEnclosures(); }; GoogleReaderFixer.prototype.fixEnclosures = function () { var nodes, o, img, src; nodes = document.evaluate("//a[span[@class='view-enclosure']]", document, null, XPathResult.UNORDERED_NODE_SNAPSHOT_TYPE, null); //alert('count='+nodes.snapshotLength); if (nodes) { for (var i = 0; i < nodes.snapshotLength; i++) { o = nodes.snapshotItem(i); div = document.createElement('div'); div.className = "item-pict"; img = document.createElement('img'); div.appendChild(img); img.src = o.href; var p = o.parentNode.parentNode; p.parentNode.replaceChild(div, p); } } } //instantiate and run /*window.addEventListener("load", function() { window.setTimeout(function(){ new GoogleReaderFixer(); }, 500); }, false);*/ document.getElementById('entries').addEventListener('DOMNodeInserted', function () { new GoogleReaderFixer(); }, true); })(); // Initiate custom CSS function function GM_addStyle(css) { var parent = document.getElementsByTagName("head")[0]; if (!parent) { parent = document.documentElement; } var style = document.createElement("style"); style.type = "text/css"; var textNode = document.createTextNode(css); style.appendChild(textNode); parent.appendChild(style); } // Custom CSS interface styling GM_addStyle(" \ a { color: #0022aa !important } \ a.entry-title-link { color: #4266A9 !important; } \ a:hover .tree-item-action-container, .scroll-tree li a.menu-open { background-color: #E5E5FF !important; } \ a.tree-link-selected .name { color: #D14836 !important; font-weight: bold !important; } \ .card { margin-left: 10px !important; padding-right: 8px !important; -webkit-border-radius: 6px !important; -moz-border-radius: 6px !important; border-radius: 6px !important; -webkit-box-shadow: 4px 4px 8px #999 !important; -moz-box-shadow: 4px 4px 8px #999 !important; box-shadow: 4px 4px 8px #999 !important; } \ .cards { border-top: 2px solid #ccc !important; } \ .cards .entry { padding: 20px 9px 0 3px !important; } \ .card-bottom { margin-left: -14px !important; margin-right: -9px !important; } \ .collapsed { line-height:2.2ex !important; padding:0px 0 !important; } \ .display-none { display:none !important; } \ .entry .star { height: 15px !important; margin-right: -3px !important; } \ .entry .entry-body, .entry .entry-title, .entry .entry-likers { max-width: 960px !important; } \ .entry .entry-actions .sharebox { background: transparent url(/reader/ui/3904077461-entry-action-icons.png) no-repeat !important; background-position: -129px -256px !important; padding: 1px 0 1px 15px !important; margin: 0 16px 0 10px !important; white-space: nowrap !important; } \ .entry-body a { color: #4266A9 !important; } \ .entry-icons { top:0 !important } \ .entry-title { max-width:800px !important } \ .entry-source-title { top:2px !important } \ .entry-secondary { top:1px !important } \ .entry-main .entry-original { top:4px !important } \ .folder .folder .folder-toggle { margin-left:13px !important } \ .folder .sub-icon, .folder .folder>a>.icon { margin-left:27px !important } \ .folder .folder>ul .icon { margin-left:34px !important } \ .folder .folder .name-text { max-width:160px; !important } \ .folder-icon, .sub-icon, .tag-icon { opacity: .8 !important; } \ .goog-flat-menu-button, #lhn-add-subscription, .goog-button-base-inner-box, .jfk-button { height: 22px !important; line-height: 22px !important } \ .goog-button-base-content { padding-top: 3px !important } \ .goog-menuitem, .goog-tristatemenuitem, .goog-filterobsmenuitem { padding: 3px 7em 3px 30px !important; } \ .goog-menuitem-highlight, goog-menuitem-hover { padding-top: 2px !important; padding-bottom: 2px !important; } \ .jfk-button-primary, .jfk-button-action { background: #95A9D6 !important; background-image: -webkit-linear-gradient(top,#A7B7DD,#95A9D6) !important; border-color: #708AC7 !important; } \ .jfk-button-primary.jfk-button-hover { background-color: #E2786E !important; background-image: -webkit-linear-gradient(top,#E78C84,#E2786E) !important; border: 1px solid #E45D4E !important; } \ .jfk-button-action.jfk-button-hover { background-color: #E2786E !important; background-image: -webkit-linear-gradient(top,#E78C84,#E2786E) !important; border: 1px solid #E45D4E !important; } \ .jfk-textinput { height: 20px !important } \ .lhn-section { line-height: normal !important ; background: transparent !important; } \ .lhn-section-primary { line-height: 20px !important; font-size: 9pt !important; } \ .overview-section-header { color: #4266A9 !important; font-size: 165% !important; font-weight: bold !important; line-height: 37px !important; padding: 0 .7em !important; } \ .previewLink { background: transparent url(/reader/ui/3904077461-entry-action-icons.png) no-repeat !important; background-position: -208px -416px !important; padding: 2px 0px 0px 15px !important; margin-right: 8px !important; white-space: nowrap !important; text-decoration: none !important;} \ .read a.entry-title-link { color: #999 !important; } \ .read .card { border: #ccc solid 2px !important; background: transparent !important; } \ .samedir { background-color: white !important; } \ .scroll-tree { font-size: 9pt !important; } \ .scroll-tree .folder-icon { background-position: -47px -15px !important; } \ .scroll-tree .icon, .scroll-tree .favicon { width: 17px !important; height: 17px !important; } \ .scroll-tree li.sub { height: 18px; padding: 1px 0 !important; } \ .scroll-tree li .cursor { background-color: #E5E5FF !important; } \ .scroll-tree li a:hover { background-color: #E5E5FF !important; } \ .scroll-tree li { background: transparent !important; margin: 2px 0 !important; } \ .scroll-tree li a.tree-link-selected .name, .scroll-tree li a.tree-link-selected:hover .name { color: #D63C2E !important; } \ .section-minimize { top: 0; left: 0px !important } \ .selectors-footer { margin-bottom: 0 !important ; padding-bottom: 5px !important } \ .selector:hover, #lhn-selectors .selector:hover { background-color: #F0F3F9 !important; } \ .tree-link-selected { border-left: 3px solid #D14836 !important; background-color: #E5E5FF !important; } \ .unread-count { padding: 0 0 0 8px !important; font-size: 100% !important; font-weight: bold !important; color: #666 !important; } \ #chrome-title { padding-left: 5px !important; color: #355089 !important; font-size: 18px !important; line-height: 20px !important; } \ #chrome-title a { color: #355089 !important; font-size: 17px !important; } \ #current-entry .card, .card { border: 2px solid #95A9D6 !important; } \ #current-entry .entry-title-link { color: #4266A9 !important; } \ #entries { padding:0 !important; } \ #entries .collapsed .entry-title { color: #4266A9 !important; } \ #entries .entry, #entries.list .entry-container { background: transparent !important } \ #entries.list .collapsed .entry-icons, #entries.list .collapsed .entry-main .entry-original { margin-top: 5px; } \ #entries.list .collapsed .entry-secondary { color: #333 !important; } \ #entries.list .collapsed .entry-main .entry-source-title { color: #333 !important; font-weight: bold !important; } \ #entries.list #current-entry.expanded .entry-actions { border-bottom-width: 0px !important; } \ #entries.list .entry .collapsed { border-width: 0px 0 !important; } \ #home-section { font-size: 9pt !important; } \ #lhn-add-subscription-section { height:35px !important; } \ #lhn-selectors { font-size: 9pt !important; } \ #lhn-add-subscription, #viewer-top-controls-container { margin-top:-13px !important; } \ #lhn-friends { font-size: 9pt !important; } \ #lhn-recommendations { font-size: 9pt !important; } \ #logo { margin-top: -13px !important; height: 30px !important; background: url(data:image/png;base64,R0lGODdhigAiAP8AAP7+/vHs8ezz+Obn5wQEBC8vL/Py7E5OTkFdxlFrykllyZSj3qi25dbX1zFPwnSJ1W9vb9zi9WyE1c/Qz8fHx7i4uThVw8PL7Obq932U2KmpqXySfdvj2bfD6dTb8jli2szV197x4lt1zLO95/CSBObi3Fh513iY6J+z5SZGvUNt4pmr5CpLwpebpmR70NXTzAB5CaGu4rWzrQCCDMnR7ZuhtxqyNJCf3fe4tMWMjNzj5nyOe/Xs6tDOx4mJidfa4ye9OFNru7zC0OPy5IiTuZi08szV8mF6zTlm4Yej6pmZmsjFva6zwmR3tnWGukl15fjIWOLe48OQTPbm2PnSd/2tAv2xCEVr1+cXJ9wLJdcBGq6zr8gEHoyRp8VXWq2MjNhTXfvaiLFYYem0QmhzpnF6odVKV9M1RbW5xYiZx2eR7Ni7uU5xzvXRzDFPvD1hy/bIxhw8ujhUuFyF6GaK5g0wty1RyoaZ2qa98S1U0bvEx3eLyNh4e6coOuu4U8UZLpeKisOqidx5g/HOgu03OvCHhPEsMpteZckbMdwjNPrsyf/RWexoYsumaK9wdO1zdfR8eaOHcpdjasnN2uducVdopfSqpc2zrwMms7QEHupST+PQ0MWurOSqq/W2q7OrrUNevMS5uF1xu0tw29eLj/OUk+mGjqatwfu6KK7B8jVd1LVGUqiqs5HWmPEbJsi2lcmWlsw0P6cwQNYwPpgyP+CgQDLEQckpOfbW1Q9vGQacFd6SGcQKI+kPInOc8Xig8s5zCwxeEH6i6qSz3rdxL+ivLhipL/67NvEzNsZ6LLASKPMpLs2ILidrLrzGvXqAmaCbmrajnbWcnde7k7zbwbeHiU9ov7WjjsywjPSinszArYWNrfLcrvbjtZeetTNMqtehoCI5oK+wvTxTqdjCo/ZXVMFMVKZ7V7yOXPW/V5JKUvbCS/dLR+hOTK9IUuVJUc2gXmPAb+nHx+d7gXfYhHirgWeTbFTPY/lnYN9XZOVZathoZgAAAAAAAAAAAAAAACwAAAAAigAiAAAI/wABCBwoEINBAQQTKlzIsOHAIa3o3YsXwKHFixgzamSIociJExke0EnSIcLGkwoHtLKly14UlDBjyhwooIgJJzWYoGGSJsgVEycGzNw4AMiMHUKHKl26UIAvNi16lABgoGqDNA7GMWF6MYTRDRW5wmRAQ6xAAb+uKHmBMOGAJi1emG045GvYuRoV3JiLRwWZJQwFDCjR9mTVk3VngMW7McECsxHUvGmRNOMUT6UglWqzUBEVKFAWdTOg0EMqPB1SFUGBIfFigbiyFYJkiQdjhY7N4nkCSsZGHIxycIIFxhClqQPD+HnVQ9sYK+uQA8CQ5EQaEypGNakxwKvisJ4Ywf+6xIeQpk0KIzAQsGCEQCMiECRgQJCGCwUKJHggyCABAhEX5AaAADcogMADGAzEQAQXLFCYQ8I8EQRgGeGgiTQgVMSBGb2AkVQYxXySoQBRjFHFGFMJMMcePfDwggkfOCFXCDbAsBgO7UQzoiBarFKZQB2kcIQCMQBwAQsPMHBHCisIdEEKLjCAQgIOmATAAnFkwEAGCNTRJAAuILDClAi0ZUECCUjwYEN0qBAEBZaV44Ued62RSBY5AKAIKlIIQRA3VpAQCABFXEEZkCog4OcQNYJlAD5zCgQHGLIcAkJCF9RRpEAJ7OWkAwlKkAFBegEQQQruCXTqYyNYUFinAjn/oMCaDs2hggIUXmQJMmLoQFAA72jhjg6DWIHODwQZgAoJUnCgxgc1kAZAAE/Y0QIANNoIQBvseDEBDpTsk0MFGSbUgQMDRYCAlQIp4B5CARixnwQSALACAgnlJip/CQhkAX0anaDCGzU01BZChbjSa0KPaNFHD4tYIQWyBEFBQjITPEvEXXS40YIA2e4AAA6GmJMDKZyAEMJdBLU6kBFxIIAffl4O+IADDrCgQBwPAJDBEfk+dkQKBuLHgqsAIABwRiuoEKNt5j7w0QMP/PCIK6tcSpApXNBCARRVSNFAQlSQQMwENyAhwtgCIVFJBQAwCoPIOCwTSwWCOdSBBQPR/5DCAiigEEMMDFQkgQMXYMCgBfU+4EJCCjwmwnwxCE6WQEpvFAGMCvhJkAA0oHEKEiyQwcE8WNwSSkJcq9MAFVXskqtAZZ8DwgAi5KFfBEk0oYFQ2W4gQBuEYJEDrZiiK5AHFrDsgQACIE7QA/VmIELQYI5K0H5JL53RMKp8YMJLCRmwuQNl8LDJndWERZo+mQCiwxQkVNFIggMdAww0QkVBRBMSmEMQZOArbDWqIvnAwizWYBGXnSVzCBEAAjoQPe4JRAT1OteDEPCYBSiAIB7EnPcwYoAH2OEDVVNIAEyQgvQBgBRcQAQDJfUHSVCgItMgAQnIkRwSRMJzVCmBwP/eNJAQGOM7AJAHIrJwhk7YZgpwkNZAWlWYG1RJIBlAWgJEEMEFYKJnElSTzTDRpAg44A7LY8FjJDhCjARgD27IwyhQ8CAjKCAcZUhRDpTxBz50QhBniAQa7vIKZpDAD4NIRy2uIQSWAaADH0CCCShGjVwEoxn1qMgl+pAJXpzBDGYAhyMv8EGCuIAF/nFAWQDgAQQ4IAFnSkDPWGmBM1ngCAjY1JHkk4JZAiABHRjQSQIgDlHIwQ1vOMIDMrAHEZDhGRq4ywu+IAZHfCEU5SIICAIBj0ZgQwg6uIsRqpMBUSDgA2yYxLQmsIUtOCMpHPiCJA7hiE/8wJHQUwgNAPf/IAGMoD1noQkDFnCBARUmACgg6OdmUoIKsKIL2yBCTnrQgBI4EgADGIAALjoQgwTgLgJIAhuIwIQJvOAU1siDCH7UzwB0h6O3ceMB4BSAmW6kKjg1APJmUgRVdKEHOhXIJBQgB7jFlDE1ZRsBtnBUzfEmmgnJwDeM2lSGaOAAELghACYAgQMoQVocOMAAlACBpAQAAmPbAQEKMFMlaEAgXK2qQjCABGhJUSASqMTs5HoWCBTgrwWAmw8ACwGBUIAABzgAYgUyAALgDQJrLYAeAFEAgXSVrwm5gx2ukKoBrSAIrPgRZhsLp2mxTAYEqMgEHAuABhCAA9NiLQAI4JvW4hZAKAWoLWYFYgAiyOGV9HKBEyoA290S5AAF8MFb4dpVAhBAKKsVCgcIMAGMsjYABNAAQgxwAMoUAKZVDcALatAFInijAg0AL1/JytZpsdUHkIUuAcbm2uo2Fm7Y1YC0fHAA+BpXITlVr3Gx2wANEEAgFUgtAA4L2/rGFr/ZHUgA/lra/1r4Ihxga1crm+HEKlYoh6UvAeDU2Noi9wDVBYBfBXzhFl+1AGUdEAVgLIPv2lYoAyjA2Cac4gEgl20+EFmLh7zbpBL5yHLdQWWRzOSYaoACO22ylKdM5Spb+cpKCQgAOw==) no-repeat !important; } \ #main { background: #fff !important } \ #nav { width: 270px !important;} \ #overview { padding-right: 20px !important; margin-right: 2em !important; border-right: 1px solid #EBEBEB !important; } \ #overview .title a { color: #5D80C0 !important; font-size: 80% !important; } \ #overview-selector, #lhn-selectors .selector, .folder .name.name-d-0, \ #reading-list-unread-count { line-height: 15px !important; margin-top: 0 !important } \ #reading-list-selector .label { display:inline !important } \ #recent-activity .recent h4 a { color: #5D80C0 !important; margin: 0 !important; font-weight: bold !important; font-size: 120% !important; } \ #recommendations-tree .sub-icon { background-position: -31px 0px !important; } \ #scrollable-sections { padding-bottom: 12px !important; overflow: auto !important; border-right: 1px #ebebeb solid !important ; background: transparent !important; } \ #scrollable-sections-holder { border-right: #E5E5FF solid 1px !important;} \ #search { padding:8px 0 !important; } \ #sections-header { height: 55px !important; margin-bottom: 1em !important; } \ #sub-tree-header { padding-left: 15px !important; } \ #title-and-status-holder { padding:0.3ex 0 0 0.5em !important; background: #DEDEDE !important ; margin-right: 0 !important } \ #top-bar { height:40px !important; background: #fff !important } \ #viewer-entries-container { padding-bottom: 12px !important; } \ #viewer-header { background: transparent !important; height:35px !important; } \ ::-webkit-scrollbar-thumb { background-color: rgba(0, 0, 0, .1) !important; } \ ::-webkit-scrollbar-thumb:hover { background-color:rgba(0,0,0,.2) !important; box-shadow:inset 1px 1px 1px rgba(0,0,0,.25) !important; } \ ::-webkit-scrollbar { width: 22px !important; } \ \ "); // Google is trying to include the +1 experience into all their services, the reality is that the +1 calls that are made // by the current Google Reader interface are excessive and degrade user experience, especially speed and smooth scrolling. // It is disabled by default but if you wish to enable it, you can comment out ' // ' the line below. GM_addStyle(".item-plusone {display: none !important;}"); // Call the JQuery library for the post preview and slide out tab (coming soon) function addJQuery(callback) { var script = document.createElement("script"); script.setAttribute("src", "https://ajax.googleapis.com/ajax/libs/jquery/1.6.4/jquery.min.js"); script.addEventListener('load', function () { var script = document.createElement("script"); script.textContent = "(" + callback.toString() + ")();"; document.body.appendChild(script); }, false); document.body.appendChild(script); } // Main function to enable collapsable element keypress events function keypress() { jQuery.noConflict(); jQuery(document).bind('keydown', function (e) { // pressing 'shift w' will open and close the search bar if (e.target.nodeName.toLowerCase() != 'input' && e.shiftKey && e.keyCode == 87) { jQuery("#top-bar").toggleClass("display-none"); } // pressing 'shift q' will open and close the Google bar if (e.target.nodeName.toLowerCase() != 'input' && e.shiftKey && e.keyCode == 81) { jQuery("#gb").toggleClass("display-none"); } // pressing 'shift e' will open and close the header bar if (e.target.nodeName.toLowerCase() != 'input' && e.shiftKey && e.keyCode == 69) { jQuery("#title-and-status-holder").toggleClass("display-none"); } }); } addJQuery(keypress); // Setup and enable previews function getFirstElementMatchingClassName(root, tag, classN) { var elements = root.getElementsByTagName(tag); var i = 0; while (elements[i] && !elements[i].className.match(classN)) { i++; } return ((!elements[i]) ? null : (elements[i])); } function getElementsByClassName(root, tag, classN) { var elements = root.getElementsByTagName(tag); var results = new Array(); for (var i = 0; i < elements.length; i++) { if (elements[i].className.indexOf(classN) > -1) { results.push(elements[i]); } } return (results); } function findParentNode(el, tag, classN) { el = el.parentNode; if (arguments.length == 3) { // Find first element's parent node matching tag and className while (el.nodeName.toLowerCase() != 'body' && (el.nodeName.toLowerCase() != tag || (el.className != classN && el.className.indexOf(classN + ' ') == -1))) { el = el.parentNode; } return ((el.nodeName.toLowerCase() != 'body') ? el : false); } else { // Find first element's parent node matching tag while (el.nodeName.toLowerCase() != 'body' && el.nodeName.toLowerCase() != tag) { el = el.parentNode; } return ((el.nodeName.toLowerCase() != 'body') ? el : false); } } function addStyles(css) { var head = document.getElementsByTagName('head')[0]; if (head) { var style = document.createElement('style'); style.type = 'text/css'; style.innerHTML = css; head.appendChild(style); } } function catchEntryAdded(e) { var el = e.target; if (el.nodeName == 'DIV' && el.className.indexOf('entry') > -1) { if (el.className.indexOf('entry-actions') > -1) { // Expanding article in list view addPreviewButton(el); } else if (getFirstElementMatchingClassName(el, 'div', 'card-bottom')) { // Adding article in expanded view addPreviewButton(getFirstElementMatchingClassName(el, 'div', 'entry-actions')); } } } function addPreviewButton(el) { // Top link var entry = findParentNode(el, 'div', 'entry'); var link = getFirstElementMatchingClassName(entry, 'a', 'entry-title-link'); //link.addEventListener('click', previewMouseClick, false); link.addEventListener('click', function (e) { if (!e.ctrlKey) { previewMouseClick(e); } }, false); // Bottom button var preview = document.createElement('span'); preview.className = 'item-preview preview link'; preview.innerHTML = 'Preview'; el.appendChild(preview); preview.addEventListener('click', previewMouseClick, false); } function calcEntryIndex(e) { var index = 0; while (e.previousSibling) { index++; e = e.previousSibling; } return index; } function previewMouseClick(e) { var el = e.target; var entry = findParentNode(el, 'div', 'entry'); var index = calcEntryIndex(entry); preview(entry, index); e.preventDefault(); } function previewShortcut() { preview(document.getElementById('current-entry')) } function preview(entry) { var preview; // Update entry with preview mode, need to do it before scrolling, because scrolling will repaint preview button (list view only) if (entry.className.indexOf('preview') == -1) { entry.className = entry.className + ' preview'; preview = true; } else { entry.className = entry.className.replace('preview', ''); preview = false; } // Need to scroll before changing entry-body, because scrolling repaints article from scratch (list view only) scrollTo(entry); var body = getFirstElementMatchingClassName(entry, 'div', 'entry-body'); var entryBody = getFirstElementMatchingClassName(body, 'div', 'item-body'); if (preview) { // classic mode-> preview mode // hide rss item entryBody.style.display = 'none'; // iframe creation/display var iframe = getFirstElementMatchingClassName(entry, 'iframe', 'preview'); if (iframe) { // iframe already in document, display it iframe.style.display = 'block'; } else { // iframe not in document, create it iframe = document.createElement('iframe'); iframe.setAttribute('width', '100%'); iframe.setAttribute('height', '500px'); iframe.setAttribute('src', getFirstElementMatchingClassName(entry, 'a', 'entry-title-link')); iframe.className = 'preview'; body.appendChild(iframe); } // Scale article container to fullwidth body.setAttribute('style', 'max-width: 98%'); } else { // preview mode -> classic mode // hide iframe var iframe = getFirstElementMatchingClassName(entry, 'iframe', 'preview'); if (iframe) iframe.style.display = 'none'; // show rss item entryBody.style.display = 'block'; // Go back to initial width body.removeAttribute('style', ''); } } function handleKeypress(e) { // Handle a Shift-V keypress if (e.target.nodeName.toLowerCase() != 'input' && e.shiftKey && e.keyCode == 86) { previewShortcut(); e.preventDefault(); } } function getEntryDOMObject(index) { // Because of repaint, entry doesn't point to correct DOM object, we need to find entry using index var entries = document.getElementById('entries'); var i = 0; entry = entries.firstChild; while ((i++) < index) { entry = entry.nextSibling; } return entry; } function scrollTo(entry) { // Force scrolling to top of article try { // Navigate through DOM until reaching "entries" item, in order to compute current entry's top coordinate relative to entries' main container var top = 0; while (entry.id != 'entries') { top += entry.offsetTop; entry = entry.parentNode; } document.getElementById('entries').scrollTop = top; } catch (err) {} } function restyle() { // Overwrites Better GReader extension css modifications regarding entry-actions class. // Indeed, entry-actions was set to "float : right", thus div was not in document flow. // Then, clicking on preview button let entry actions div in place instead of going down automatically when iframe was added. // That's why I use here text-align: right. That has the same effect, but keeps div in document flow. // restyle() is called after document load, in order to ensure that Better GReader has already added its styles modifications var styles = document.getElementsByTagName('head')[0].getElementsByTagName('style'); var i = 0; while (i < styles.length) { if (styles[i].innerHTML.indexOf('.entry-actions { float:right !important; }') > -1) { styles[i].innerHTML = styles[i].innerHTML.replace('.entry-actions { float:right !important; }', '.entry-actions { text-align: right; !important; }'); } i++; } } function init() { restyle(); addStyles('span.item-preview { background: url("data:image/gif,GIF89a%10%10%D5%13%D8%D8%D8%FA%FA%FA%CB%CB%CB%C8%C8%C8%D2%D2%D2%BA%BA%BA%C6%C6%C6%A1%A1%A1%9C%9C%9C%BD%BD%BD%C9%C9%C9%AB%AB%AB%F4%F4%F4%BF%BF%BF%FC%FC%FC%DB%DB%DB%AD%AD%AD%FF%FF%FF%CC%CC%CC!%F9%04%01%13%2C%10%10%06I%C0%89pH%2C%1A%8F%C8d%F1!i%3A%9F%8F%E1%03B%ADZ%A9%D1%89%04%12%E9z%BF%10%89p%FB-G%C2c%AE%D9%8B%D6%AA%03_%F8Y%EC%8E%C8%E3%F3%F4%9AM\'%7B%1D%0E%60tW%85%10RO%8A%12YJ%8E%8EA%3B") no-repeat; padding-left: 16px; } div.entry.preview span.item-preview { background: url("data:image/gif,GIF89a%10%10%A2%05%D8%D8%D8%DB%DB%DB%AD%AD%AD%CC%CC%CC%FE%9A%20%FF%FF%FF!%F9%04%01%05%2C%10%10%03%3BX%BA%DC%FE0%B60%AA%BDa%05%C1%BB%E7Y1%08Di%9E%C2%A0%8C%A6%D7%AA%22Y%CA2%91%AE%B5%3B%C3%EC%7C%EE%B8%D6%CF%C6%AB%0D%89%0A%C0g)h.%D0AHB%A5%26%3B") no-repeat; padding-left: 16px; }'); } document.body.addEventListener('DOMNodeInserted', catchEntryAdded, false); document.addEventListener('keydown', handleKeypress, false); window.addEventListener('load', init, false); // Regularly purge elements from memory to speed up browsing experience var intPurgeTimeout = 5000; // set the timeout in ms to run the check for elements to purge function purgeRead() { var intPastRead = 10; // how many old elements to keep var objCurrent = document.getElementById('current-entry'); if (objCurrent != undefined) { var intPast = 0; var objP = objCurrent; while (intPast <= intPastRead && objP != undefined) { objP = objP.previousSibling; intPast++; } while (objP != undefined) { var arrClasses = objP.className.split(' '); var intClasses = arrClasses.length; var boolPurged = false; var boolRead = false; for (var i = 0; i < intClasses; i++) { if (arrClasses[i] == 'read') { boolRead = true; } if (arrClasses[i] == 'purged') { boolPurged = true; } } if (boolRead == true && boolPurged == false) { console.log('Google Reader Skim Purge: Removing item with class of "' + objP.className + '"'); objP.className = objP.className + ' purged'; //var objRemoves = objP.childNodes; var objRemoves = objP.getElementsByClassName('entry-body'); for (var i = objRemoves.length; i > 0; i--) { objRemoves[i - 1].parentNode.style.height = objRemoves[i - 1].parentNode.clientHeight + 'px'; objRemoves[i - 1].innerHTML = 'Entry body removed by Google Reader Skim Purge'; objRemoves[i - 1].parentNode.removeChild(objRemoves[i - 1]); } } objP = objP.previousSibling; } } } console.log('Google Reader Skim Purge: Initilizing purger to ' + intPurgeTimeout + ' millisecond interval.'); setInterval(purgeRead, intPurgeTimeout); // Auto update notification for Firefox browser if (navigator.userAgent.toLowerCase().indexOf('firefox') > -1) { var AnotherAutoUpdater = { // Config values, change these to match your script id: '117298', // Script id on Userscripts.org days: 1, // Days to wait between update checks // Don't edit after this line, unless you know what you're doing ;-) name: /\/\/\s*@name\s+(.*)\s*\n/i.exec(scr_meta)[1], history: /\/\/\s*@history\s+(.*)\s*\n/i.exec(scr_meta)[1], version: /\/\/\s*@version\s+(.*)\s*\n/i.exec(scr_meta)[1].replace(/\./g, ''), time: new Date().getTime(), call: function (response) { GM_xmlhttpRequest({ method: 'GET', url: 'https://userscripts.org/scripts/source/' + this.id + '.meta.js', onload: function (xpr) { AnotherAutoUpdater.compare(xpr, response); } }); }, compare: function (xpr, response) { this.xversion = /\/\/\s*@version\s+(.*)\s*\n/i.exec(xpr.responseText); this.xname = /\/\/\s*@name\s+(.*)\s*\n/i.exec(xpr.responseText); this.xhistory = /\/\/\s*@history\s+(.*)\s*\n/i.exec(xpr.responseText); if ((this.xversion) && (this.xname[1] == this.name)) { this.xversion = this.xversion[1].replace(/\./g, ''); this.xname = this.xname[1]; this.xhistory = this.xhistory[1]; } else { if ((xpr.responseText.match("the page you requested doesn't exist")) || (this.xname[1] != this.name)) GM_setValue('updated_' + this.id, 'off'); return false; } if ((+this.xversion > +this.version) && (confirm('A new version of the ' + this.xname + ' user script is available. Do you want to update?\n\nChangelog:\n' + this.xhistory + ''))) { GM_setValue('updated_' + this.id, this.time + ''); top.location.href = 'https://userscripts.org/scripts/source/' + this.id + '.user.js'; } else if ((this.xversion) && (+this.xversion > +this.version)) { if (confirm('Do you want to turn off auto updating for this script?')) { GM_setValue('updated_' + this.id, 'off'); GM_registerMenuCommand("Auto Update " + this.name, function () { GM_setValue('updated_' + this.id, new Date().getTime() + ''); AnotherAutoUpdater.call(true); }); alert('Automatic updates can be re-enabled for this script from the User Script Commands submenu.'); } else { GM_setValue('updated_' + this.id, this.time + ''); } } else { if (response) alert('No updates available for ' + this.name); GM_setValue('updated_' + this.id, this.time + ''); } }, check: function () { if (GM_getValue('updated_' + this.id, 0) == 0) GM_setValue('updated_' + this.id, this.time + ''); if ((GM_getValue('updated_' + this.id, 0) != 'off') && (+this.time > (+GM_getValue('updated_' + this.id, 0) + (1000 * 60 * 60 * 24 * this.days)))) { this.call(); } else if (GM_getValue('updated_' + this.id, 0) == 'off') { GM_registerMenuCommand("Enable " + this.name + " updates", function () { GM_setValue('updated_' + this.id, new Date().getTime() + ''); AnotherAutoUpdater.call(true); }); } else { GM_registerMenuCommand("Check " + this.name + " for updates", function () { GM_setValue('updated_' + this.id, new Date().getTime() + ''); AnotherAutoUpdater.call(true); }); } } }; if (self.location == top.location && typeof GM_xmlhttpRequest != 'undefined') AnotherAutoUpdater.check(); }
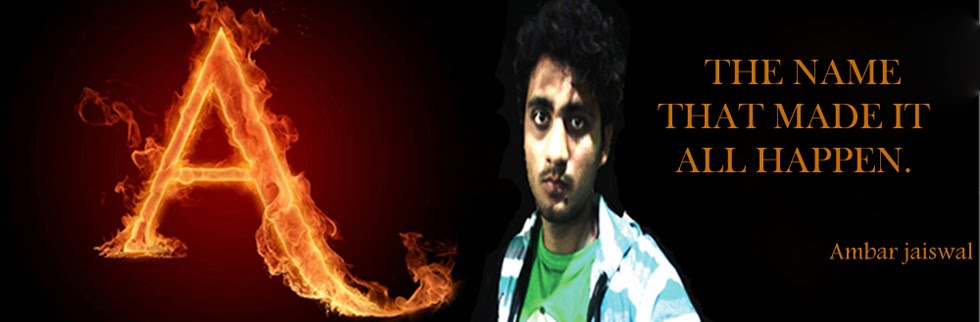
Mozilla add on,User script,Grease Monkey Script, greasemonkey userscripts, updater userscripts mafia wars userscripts mafia wars autoplayer userscripts mafia wars wall userscripts scripts userscripts travian greasemonkey greasemonkey download greasemonkey facebook greasemonkey tutorial greasemonkey youtube greasemonkey travian greasemonkey chrome greasemonkey mafia wars greasemonkey mafia wars autoplayer
Thursday, November 24, 2011
Google Reader Sanity
Subscribe to:
Post Comments (Atom)
0 comments:
Post a Comment