// ==UserScript== // @name YouTube - Back to the Old! // @version 2.0 // @description Return YouTube to what it used to be + MORE! // @run-at document-start // @match http://www.youtube.com/ // @match http://www.youtube.com/* // @match https://www.youtube.com/ // @match https://www.youtube.com/* // ==/UserScript== var YTBTTO = { Origin: window.location.protocol + "//www.youtube.com/", Redirect: "index_ajax", Config: "old:config", Logo: "http://i.imgur.com/00tbO.png", Settings: {}, Defaults: { "NewHomepage": 0, "NewColorScheme": 0, "UseRedirect": 1, "CollapseModules": 0 }, ApplySettings: function() { for (var i in YTBTTO.Defaults) { var Setting = localStorage.getItem("YTBTTO-" + i); if (Setting !== "NaN" && Setting !== null && Setting !== undefined) { YTBTTO.Settings[i] = parseInt(Setting); } else { YTBTTO.Settings[i] = YTBTTO.Defaults[i]; localStorage.setItem("YTBTTO-" + i, YTBTTO.Settings[i]); } } }, DisplaySettings: function() { for (var i in YTBTTO.Settings) { if (YTBTTO.Settings[i]) { document.querySelector("form#" + i + " #safety-mode-on").checked = 1; } else { document.querySelector("form#" + i + " #safety-mode-off").checked = 1; } } }, ApplyDefaults: function() { for (var i in YTBTTO.Defaults) { YTBTTO.Settings[i] = YTBTTO.Defaults[i]; YTBTTO.DisplaySettings(); } }, Save: function() { for (var i in YTBTTO.Settings) { var Checked = document.querySelector("form#" + i + " #safety-mode-on").checked; YTBTTO.Settings[i] = Checked ? 1 : 0; localStorage.setItem("YTBTTO-" + i, YTBTTO.Settings[i]); } window.location.reload(); }, Run: function() { YTBTTO.ApplySettings(); if (!YTBTTO.Settings["NewHomepage"] && window.location.href === YTBTTO.Origin) { var HTML = document.querySelector("html"); var Request = new XMLHttpRequest(); Request.open("GET", YTBTTO.Origin + YTBTTO.Redirect, false); Request.send(null); if (Request.status == 200) { HTML.innerHTML = Request.responseText; } } if (YTBTTO.Settings["UseRedirect"] && window.location.href === YTBTTO.Origin) { window.location = YTBTTO.Origin + YTBTTO.Redirect; } if (window.location.href === YTBTTO.Origin + YTBTTO.Config) { var HTML = document.querySelector("html"); HTML.innerHTML = Pages[YTBTTO.Config]; } window.addEventListener("load", function() { if (!YTBTTO.Settings["NewHomepage"] && window.location.href === YTBTTO.Origin) { HTML.removeChild(document.querySelectorAll("head")[1]); HTML.removeChild(document.querySelectorAll("body")[1]); } if (!YTBTTO.Settings["NewColorScheme"]) { var LogoImg = document.getElementById("logo"); if (LogoImg) { LogoImg.src = YTBTTO.Logo; } } if (YTBTTO.Settings["UseRedirect"]) { var LogoLink = document.getElementById("logo-container"); if (LogoLink) { LogoLink.href = YTBTTO.Origin + YTBTTO.Redirect; } } if (YTBTTO.Settings["CollapseModules"]) { var RecTitle = document.querySelector("#REC-titlebar .fm-title-underlined"), RecContent = document.getElementById("REC-content"); if (RecTitle && RecContent) { RecTitle.innerHTML += '<span id="rec-collapse" style="cursor:pointer;color:#C70031;font-size:13px;float:right;">show</span>'; var RecCollapse = document.getElementById("rec-collapse"); RecContent.style.display = "none"; RecCollapse.addEventListener("click", function() { if (RecCollapse.innerHTML === "hide") { RecContent.style.display = "none"; RecCollapse.innerHTML = "show"; } else { RecContent.style.display = "block"; RecCollapse.innerHTML = "hide"; } }); } var RecTitleNew = document.querySelector("#video-sidebar h3"), RecContentNew = document.getElementById("recommended-videos"); if (RecTitleNew && RecTitleNew.innerHTML.indexOf("Recommended") && RecContentNew) { RecTitleNew.innerHTML += '<span id="recnew-collapse" style="cursor:pointer;color:#C70031;font-size:10px;float:right;">show</span>'; var RecCollapseNew = document.getElementById("recnew-collapse"); RecContentNew.style.display = "none"; RecCollapseNew.addEventListener("click", function() { if (RecCollapseNew.innerHTML === "hide") { RecContentNew.style.display = "none"; RecCollapseNew.innerHTML = "show"; } else { RecContentNew.style.display = "block"; RecCollapseNew.innerHTML = "hide"; } }); } } // Config var Nav = document.getElementById("masthead-user"), NavBox = document.getElementById("masthead-search-bar-container"), ConfigLink = YTBTTO.Config, ConfigColor = "#C70031"; if (Nav) { Nav.innerHTML += '<span class="masthead-link-separator">|</span><a href="/' + ConfigLink + '" style="color:' + ConfigColor + ';">Config</a>'; } if (NavBox) { NavBox.style.paddingRight = "35px"; } if (window.location.href === YTBTTO.Origin + YTBTTO.Config) { HTML.removeChild(document.querySelectorAll("head")[1]); HTML.removeChild(document.querySelectorAll("body")[1]); HTML.removeChild(document.querySelectorAll("style")[document.querySelectorAll("style").length - 1]); YTBTTO.DisplaySettings(); document.getElementById("save").addEventListener("click", function() { YTBTTO.Save(); }); document.getElementById("defaults").addEventListener("click", function() { YTBTTO.ApplyDefaults(); }); document.getElementById("close").addEventListener("click", function() { window.location = YTBTTO.Origin; }); } // Error if (window.location.href === YTBTTO.Origin || window.location.href === YTBTTO.Origin + YTBTTO.Redirect) { var ErrorBox = document.getElementById("error-box"); ErrorContent = document.querySelector(".yt-alert-content"); if (ErrorBox && ErrorBox.parentNode && ErrorContent && ErrorContent.parentNode && ( ErrorContent.innerHTML.indexOf("Authorisation Error.") || ErrorContent.innerHTML.indexOf("Authorization Error.") )) { ErrorBox.parentNode.removeChild(ErrorBox); } } if (!YTBTTO.Settings["NewColorScheme"]) { var Color = "white" // All var Body = document.body, Page = document.getElementById("page"); if (Body) { Body.style.cssText += "background:" + Color; } if (Page && Page.parentNode) { Page.style.cssText += "background:" + Color; } // Watch var WatchRelated = document.getElementById("watch-related-container"), WatchSidebarFoot = document.querySelector(".watch-sidebar-foot"); if (WatchRelated && WatchRelated.parentNode) { WatchRelated.style.cssText += "background:" + Color; } if (WatchSidebarFoot && WatchSidebarFoot.parentNode) { WatchSidebarFoot.style.cssText += "background:" + Color; } } }, false); } }; if (window.location.href === YTBTTO.Origin + YTBTTO.Config) { var Pages = { "old:config": '\ <html>\ <head>\ <title>YouTube - Back to the Old! - Config</title>\ <link rel="stylesheet" href="//s.ytimg.com/yt/cssbin/www-refresh-vflMpNCTQ.css">\ <link rel="stylesheet" href="//s.ytimg.com/yt/cssbin/www-the-rest-vflNb6rAI.css">\ <link rel="stylesheet" href="//s.ytimg.com/yt/cssbin/www-home-vflzcBbtM.css">\ <style>\ #logoconfig{opacity: 0.7;position: relative;left: 58%;font-size: 40px;}\ #logotext{opacity: 0.6;position: relative;display: inline;top: -10px;margin: 0 25px 0 25px;font-size: 25px;}\ #logobox{margin: 10px auto;width:330px;}\ #logo{display: inline;background: no-repeat url(//s.ytimg.com/yt/imgbin/www-refresh-vfl3IcHHw.png) 0 -37px;width: 93px;height: 37px;}\ .divider{width: 968px;margin: 8px auto 8px auto;border-top: solid 1px White;border-bottom: solid 1px Grey;opacity: 0.5;}\ #options{width: 980px;margin: auto;padding-left: 10px;}\ #picker-container{width: 49%;display: inline-block;}\ .picker-top{height: 55px;}\ #save{margin: 10px;}\ #close{float: right;margin-right: 15px;}\ </style>\ </head>\ <body>\ <div id="logobox">\ <a href="/"><img id="logo" src="//s.ytimg.com/yt/img/pixel-vfl3z5WfW.gif" alt="YouTube home"></a>\ <div id="logotext">Back to the Old!</div>\ <div id="logoconfig">Config</div>\ </div>\ <div class="divider"></div>\ <div id="options">\ <div id="picker-container">\ <div id="safetymode-picker" class="yt-tile-static">\ <div class="picker-top">\ <h2>New homepage layout</h2>\ <p>\ Use YouTube\'s new homepage layout, turn off for the old tiled layout.\ </p>\ </div>\ <div>\ <form id="NewHomepage" class="setting">\ <p>\ <label>\ <input id="safety-mode-on" name="safety_mode" type="radio" value="true">\ On\ </label>\ <label>\ <input id="safety-mode-off" name="safety_mode" type="radio" value="false">\ Off\ </label>\ </p>\ </form>\ </div>\ </div>\ </div>\ <div id="picker-container">\ <div id="safetymode-picker" class="yt-tile-static">\ <div class="picker-top">\ <h2>New color scheme</h2>\ <p>\ Use YouTube\'s new grey color scheme, turn off for the old white scheme.\ </p>\ </div>\ <div>\ <form id="NewColorScheme" class="setting">\ <p>\ <label>\ <input id="safety-mode-on" name="safety_mode" type="radio" value="true">\ On\ </label>\ <label>\ <input id="safety-mode-off" name="safety_mode" type="radio" value="false">\ Off\ </label>\ </p>\ </form>\ </div>\ </div>\ </div>\ <div id="picker-container">\ <div id="safetymode-picker" class="yt-tile-static">\ <div class="picker-top">\ <h2>Homepage redirect</h2>\ <p>\ Redirect to /index_ajax insted of automatically downloading it to youtube.com/\ </p>\ </div>\ <div>\ <form id="UseRedirect" class="setting">\ <p>\ <label>\ <input id="safety-mode-on" name="safety_mode" type="radio" value="true">\ On\ </label>\ <label>\ <input id="safety-mode-off" name="safety_mode" type="radio" value="false">\ Off\ </label>\ </p>\ </form>\ </div>\ </div>\ </div>\ <div id="picker-container">\ <div id="safetymode-picker" class="yt-tile-static">\ <div class="picker-top">\ <h2>Collapsible Modules</h2>\ <p>\ Allows you to hide/show youtube modules such as \'Recommended\' etc.\ </p>\ </div>\ <div>\ <form id="CollapseModules" class="setting">\ <p>\ <label>\ <input id="safety-mode-on" name="safety_mode" type="radio" value="true">\ On\ </label>\ <label>\ <input id="safety-mode-off" name="safety_mode" type="radio" value="false">\ Off\ </label>\ </p>\ </form>\ </div>\ </div>\ </div>\ <div class="divider"></div>\ <button id="save" class="yt-uix-button"><span class="yt-uix-button-content">Save</span></button>\ <button id="defaults" class="yt-uix-button"><span class="yt-uix-button-content">Defaults</span></button>\ <button id="close" class="yt-uix-overlay-close yt-uix-button yt-uix-button-primary"><span class="yt-uix-button-content">Close</span></button>\ </div>\ </body>\ </html>\ ' }; } YTBTTO.Run();
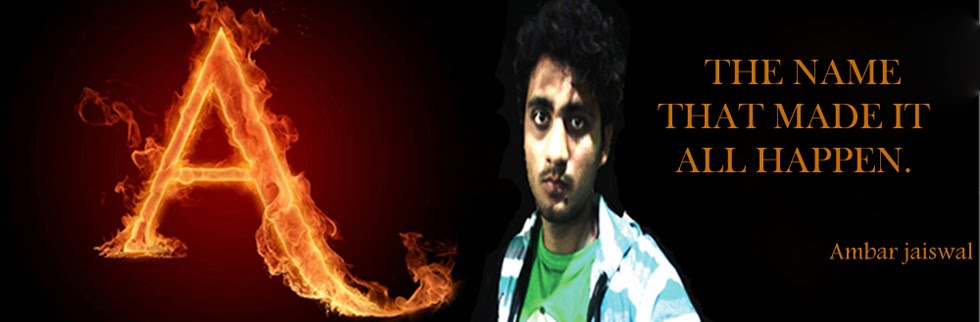
Mozilla add on,User script,Grease Monkey Script, greasemonkey userscripts, updater userscripts mafia wars userscripts mafia wars autoplayer userscripts mafia wars wall userscripts scripts userscripts travian greasemonkey greasemonkey download greasemonkey facebook greasemonkey tutorial greasemonkey youtube greasemonkey travian greasemonkey chrome greasemonkey mafia wars greasemonkey mafia wars autoplayer
Wednesday, December 7, 2011
YouTube - Back to the Old!
Subscribe to:
Post Comments (Atom)
0 comments:
Post a Comment