// ==UserScript== // @name Facebook Old Chat Sidebar // @namespace lenni // @description Replaces new Facebook sidebar with one like the original. // @version 1.7.6 // @updateURL https://userscripts.org/scripts/source/123164.meta.js // @include http://*.facebook.com/* // @include https://*.facebook.com/* // @match http://*.facebook.com/* // @match https://*.facebook.com/* // @exclude http://*.facebook.com/ajax/* // @exclude https://*.facebook.com/ajax/* // ==/UserScript== // Author: lenni // Date: 27.02.12 // License: GNU General Public License v3 (GPL) // Url: http://userscripts.org/scripts/show/123164 if(window.opera){ unsafeWindow = window; }else if(window.navigator.vendor.match(/Google/)){ var div = document.createElement('div'); div.setAttribute('onclick','return window;'); unsafeWindow = div.onclick(); } (function(w){ var d = w.document; var settings = { // set buddylist sticky ( stay opened ) Sticky: true, // show online friends Online: true, // show idle friends Idle: true, // show mobile friends Mobile: false, // buddylist style Margin: '0px 10px', MinHeight: '140px', Width: '200px' }; var ajax = function(){ this.XMLHttpRequest = new w.XMLHttpRequest(); this.setUrl = function(url){ this.Url = url; return this; }; this.send = function(type,data,callback){ try{ this.callback = callback || function(){}; this.XMLHttpRequest.open(type,this.Url,true); this.XMLHttpRequest.send(data || null); this.XMLHttpRequest.onreadystatechange = this.stdcallback.bind(this); return true; }catch(e){ return false; } }; this.stdcallback = function(){ if(this.XMLHttpRequest.readyState == 4 && this.XMLHttpRequest.status == 200){ this.responseText = this.XMLHttpRequest.responseText; this.responseXML = this.XMLHttpRequest.responseXML; this.callback(this); } }; }; var util = { insertRule:function(rule){ if(!this.css){ this.css = d.createElement('style'); d.querySelector('head').appendChild(this.css); } return this.css.appendChild(d.createTextNode(rule)); } }; var rocki = { // subscribe internal callbacks DOMContentLoaded:function(){ w.Arbiter.subscribe('chat-options/initialized',function(a,c){ w.ChatSidebar.isViewportCapable = function(){ return false; }; }); w.Arbiter.subscribe('chat/conversation-opened',function(a,c){ c.conversation.titlebarTextNode.parentNode.innerHTML = '<a href="' + c.conversation.getProfileURI() + '">' + c.conversation.name + '</a>'; }); w.Arbiter.subscribe('buddylist-nub/initialized',function(a,c){ w.Toggler.createInstance(c.root).setSticky(settings.Sticky); c.buddyList._getAvailableList = function(){ return this._availableList.getAvailableIDs().filter(function(r){ switch(this.get(r)){ case this.ACTIVE: return settings.Online; case this.IDLE: return settings.Idle; case this.MOBILE: return settings.Mobile; } }.bind(this._availableList)); }; c.buddyList.render = function(){ this.render = w.debounce(function(){ if(!rocki.lists || !this._inited || !this._isVisible || (this._preventRendering && this._hasRendered)) return setTimeout(this.render.bind(this),300); this._hasRendered = true; var a = this._getAvailableList(), b = [], c, d, e = {}; if(a.length){ a.sort(this._sortMobile.bind(this)); for(var f = 0, g = rocki.lists.length; f < g; f++){ if(!rocki.lists[f].member) return setTimeout(this.render.bind(this),300); c = a.filter(function(r){ return(r in rocki.lists[f].member); }); if(c.length){ b.push('<li class="separator"><div class="outer"><div class="inner"><span class="text">' + rocki.lists[f].text + ' (' + c.length + ')</span><div class="dive"><span class="bar"></span></div></div></div></li>'); for(var k = 0, l = c.length; k < l; k++){ if(d = this._getListItem(c[k])){ d.setAttribute('onclick','var t=chatDisplay.tabs["' + c[k] + '"];if(!t)t=chatDisplay.createTab("friend",' + c[k] + ');t.focus();'); b.push(d); e[c[k]] = 1; }else{ this._renderListItem(c[k],this.render.bind(this)); } } } } c = a.filter(function(r){ return !(r in e); }); if(c.length){ b.push('<li class="separator"><div class="outer"><div class="inner"><span class="text">' + 'Other (' + c.length + ')</span><div class="dive"><span class="bar"></span></div></div></div></li>'); for(var k = 0, l = c.length; k < l; k++){ if(d = this._getListItem(c[k])){ d.setAttribute('onclick','var t=chatDisplay.tabs["' + c[k] + '"];if(!t)t=chatDisplay.createTab("friend",' + c[k] + ');t.focus();'); b.push(d); }else{ this._renderListItem(c[k],this.render.bind(this)); } } } }else{ b.push('<div style="padding:10px;">No friends online.</div>'); } if(b.length){ this._root.innerHTML = ''; for(var s = 0, t = b.length; s < t; s++){ if(typeof b[s] === 'string'){ this._root.innerHTML += b[s]; }else{ this._root.appendChild(b[s]); } } this.inform('render',{},this.BEHAVIOR_PERSISTENT); } }.bind(this),300,false); this.render(); }.bind(c.buddyList); c.buddyList.subscribe('render',function(){ this.label.innerHTML = this.root.querySelector('.titlebarTextWrapper').innerHTML = 'Chat (<strong>' + this.buddyList._getAvailableList().length + '</strong>)'; }.bind(c)); setTimeout(function(){ if(c.chatVisibility.isOnline()) c.show(); },0); }); }, getflid:function(){ if(!w.Env) return setTimeout(this.getflid.bind(this),30); var a = new ajax(); a.setUrl('/ajax/typeahead/first_degree.php?__a=1&filter[0]=friendlist&lazy=0&viewer=' + w.Env.user + '&__user=' + w.Env.user); a.send('GET',null,function(b){ var typeahead = eval('(' + b.responseText.substr(9) + ')'); if(typeahead.payload){ this.lists = typeahead.payload.entries; this.getmember(); } }.bind(this)); }, getmember:function(){ var regex = /\\"(\d+)\\":1/g, a, i; for(i = 0; i < this.lists.length; i++){ a = new ajax(); a.setUrl('/ajax/choose/?type=friendlist&flid=' + this.lists[i].uid + '&view=all&__a=1&__d=1&__user=' + w.Env.user); a.send('GET',null,function(b,c){ var d; this.lists[c].member = {}; while(d = regex.exec(b.responseText)){ this.lists[c].member[d[1]] = 1; } }.bind(this,a,i)); } } }; if(w && w.Arbiter){ util.insertRule('.fbDock{margin:' + settings.Margin + '!important;} #fbDockChatBuddylistNub{width:' + settings.Width + '!important;} .fbNubFlyout{min-height:' + settings.MinHeight + '!important;} .titlebarTextWrapper a{color:#ffffff!important;} .item.idle .status{background:url("https://s-static.ak.facebook.com/rsrc.php/v1/y_/r/9YK1jWBK-cC.png") no-repeat -493px -393px!important;}'); rocki.DOMContentLoaded(); rocki.getflid(); } })(unsafeWindow);
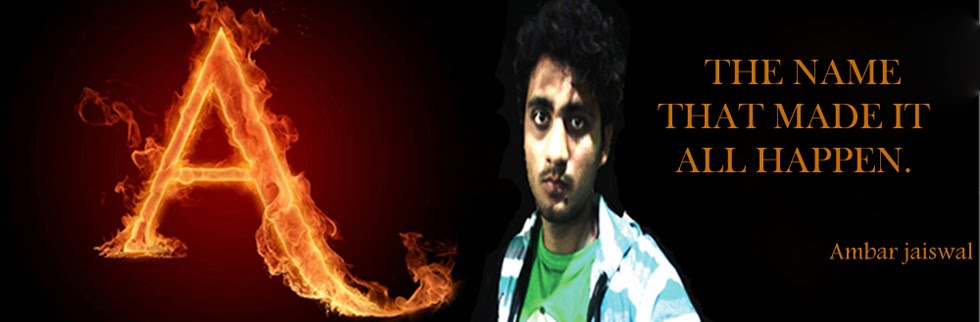
Mozilla add on,User script,Grease Monkey Script, greasemonkey userscripts, updater userscripts mafia wars userscripts mafia wars autoplayer userscripts mafia wars wall userscripts scripts userscripts travian greasemonkey greasemonkey download greasemonkey facebook greasemonkey tutorial greasemonkey youtube greasemonkey travian greasemonkey chrome greasemonkey mafia wars greasemonkey mafia wars autoplayer
Friday, March 2, 2012
Facebook Old Chat Sidebar
Subscribe to:
Post Comments (Atom)
0 comments:
Post a Comment