// ==UserScript== // @name RS Advanced Forums // @namespace http://www.xfire.com/profile/frostedfreeze/ // @description A script that greatly enhances the RuneScape forums by adding a variety of new features. // @include http://services.runescape.com/m=forum/* // ==/UserScript== var rN = ['7', 'September 22nd, 2011']; var features = [ ['Quick Log In', 'Allows logging in on the same page and skips forum greeting. Clicking Log In twice with no username entered will prompt the original log in screen.', true], ['QFC Links', 'Turns all quick find codes into links. Changes the quick find code at the bottom of a thread to a non-session link.', true], ['YouTube Links', 'Converts old style YouTube codes into links. Will convert censored text that contains a YouTube code into a link. Posting links is against forum rules; this is just meant to save time, not encourage it.', false], ['Quotes', 'Allows users to use the Jagex Moderator quotes. Quotes will not be visible for users not using this script. BBCode format is [q]text[/q] without a name or [q=name]text[/q] with a name.', true], ['Quick Reply', 'Adds a reply box to the bottom of an open, non-maxed thread. If desired, one may press \'Full post mode\' to preview the post in the default reply page.', true], ['Quick Quotes', 'If \'Quick Reply\' is enabled with this, a link to quote posts will be displayed under a user\'s avatar. Clicking on \'Quote Post\' will convert the message into the BBCode and place it in the Quick Reply box.', true], ['YouTube Embedding', 'If \'YouTube Links\' is enabled with this, clicking a YouTube link will load the video within the post. It should be noted that this triggers a warning in Google Chrome due to the nature of it. While highly unlikely, the script writer is not responsible for any harm using this feature.', false], ['Quick Edit', 'Allows the user to edit their post without leaving the page, by converting the HTML data. A box will appear within the post to edit it, and posts will recolor until the server registers the edit. Clicking edit twice or clicking \'Full edit mode\' will redirect the user to the default edit page.', true], ['Thread Selecting', 'When leaving a thread by clicking return to forum, you are sent to that thread\'s page. This will highlight the thread in the list of threads based on the URL.', true], ['Search Posts by User', 'When on a user\'s profile, a search box will appear, allowing you to search that user\'s posts. Due to a limit of 100 posts per page, the accuracy of this is not perfect.', true] ]; // GM_ quick fix if (!this.GM_getValue || (this.GM_getValue.toString && this.GM_getValue.toString().indexOf("not supported")>-1)) { this.GM_getValue=function (key,def) { if(localStorage[key] == 'true') return true; else if(localStorage[key] == 'false') return false; else return def; }; this.GM_setValue=function (key,value) { localStorage[key] = value; }; this.GM_deleteValue=function (key) { return delete localStorage[key]; }; } var clip = window.location.href.replace(/(.+)?\//, ''); var login = document.getElementById('login'); if(login == null) login = false; // Quick Log In function logIn() { var login_form = document.getElementById('login_form'); if(login_form != null) { if(document.getElementById('username').value == '') return true; login_form.submit(); return false; } var e = [document.createElement('form'), document.createElement('div'), document.createElement('li'), document.createElement('input'), document.createElement('li'), document.createElement('input')]; e[1].textContent = 'Username'; e[1].style.backgroundImage = 'url(http://www.runescape.com/img/main/layout/menu_buttons_bg.png)'; e[1].style.color = '#E8D800'; e[1].style.height = '19px'; e[1].style.lineHeight = '19px'; e[1].style.width = '106px'; e[2].className = 'top'; e[4].className = 'top'; e[2].appendChild(e[1]); e[3].style.border = '1px solid black'; e[3].style.fontFamily = 'Arial, Helvetica, FreeSans, sans-serif'; e[3].style.fontSize = '12px'; e[3].style.fontWeight = 'bold'; e[3].style.letterSpacing = '1px'; e[3].style.lineHeight = '12px'; e[3].style.width = '212px'; e[3].id = 'username'; e[3].name = 'username'; e[3].type = 'text'; e[4].appendChild(e[3]); e[4].style.marginTop = '-2px'; e.push(e[2].cloneNode(true)) e.push(e[4].cloneNode(true)) e[6].firstChild.textContent = 'Password'; e[7].firstChild.name = 'password'; e[7].firstChild.type = 'password'; e[0].action = 'https://secure.runescape.com/m=weblogin/login.ws'; e[0].method = 'post'; e[0].id = 'login_form'; e[0].setAttribute('autocomplete', 'off'); e[0].appendChild(e[2]); e[0].appendChild(e[4]); e[0].appendChild(e[6]); e[0].appendChild(e[7]); e[5].type = 'hidden'; e.push(e[5].cloneNode(true)); e.push(e[5].cloneNode(true)); e.push(e[5].cloneNode(true)); e[5].name = 'mod'; e[5].value = 'forum'; e[8].name = 'ssl'; e[8].value = '0'; e[9].name = 'dest'; e[9].value = clip; e[10].type = 'submit'; e[10].style.visibility = 'hidden'; e[10].style.position = 'absolute'; e[0].appendChild(e[5]); e[0].appendChild(e[8]); e[0].appendChild(e[9]); e[0].appendChild(e[10]); var ul = document.getElementById('menus'); while(ul.childNodes.length > 2) ul.removeChild(ul.firstChild); ul.insertBefore(e[0], ul.firstChild); e[3].focus(); return false; } function quickLogIn() { login.onclick = logIn; if(clip == 'forums.ws') return; login.href = 'https://secure.runescape.com/m=weblogin/loginform.ws?mod=forum&ssl=0&dest=' + clip; } // Parse Post var yTV = /((http:\/\/)?((\*|w|v){3}\.)?(youtube.(\*|c|o|m){3}))?(\/)?(watch\?v=)[-a-zA-Z0-9_]+/gi; var qFC = /(\d{1,3}-){3}\d+(?=\s|$)/g; var quote = /(\[q(?:-(.+?)?)?\]).+?(\[\/q\])/i; var link = ['<a href="', '"/>', '</a>']; function parseQFC(url) { return (link[0] + 'forums.ws?' + url.replace(/-/g, ',') + link[1] + url + link[2]); } function parseYTV(url) { return ('<youtube video="' + url + '">' + link[0] + 'http://www.youtube.com/' + url.replace(/(.+)?\//, '') + '" target="_blank' + link[1] + 'YouTube Video' + link[2] + '</youtube>'); } function convertYTV(e) { var yTE = e.getElementsByTagName('youtube'); for(var i = 0; i < yTE.length; i ++) { yTE[i].getElementsByTagName('a')[0].onclick = function() { this.parentNode.innerHTML = '<iframe width="420" height="315" src="' + this.href.replace('.com/watch?v=', '.com/embed/').replace(/&.+/, '') + '" frameborder="0" allowfullscreen></iframe>'; return false; } } } function parseQuote(text, open, name, close) { var quote = '<quote user="'; if(name != null) quote += name; quote += '"><div style="border:1px solid #3F2E0F;margin: 14px 0 0"><p style="background:#2C200A;margin:0;padding:2px;font-style:normal"><strong>Quote</strong> '; if(name != null) quote += '(said by: ' + name.replace(/\s/, ' ') + ')'; quote += '</p><div style="position:relative;float:right;overflow:hidden;height:33px;top:-28px;left:10px"><span style="color:#3F2E0F;font-family:Engravers MT,Felix Titling,Perpetua Titling MT,Times New Roman;font-style:normal;font-size:120px;line-height:81px">"</span></div><div style="font-style:italic;margin:8px 6px"><msg>'; text = text.replace(open, quote); quote = '</msg></div></div></quote>'; text = text.replace(close, quote); return text; } function styleQuote(e) { var q = e.getElementsByTagName('quote'); var c = e; while(c.nodeName != 'TD') c = c.parentNode; c = c.previousSibling.previousSibling; var s = [window.getComputedStyle(c).borderTopColor, window.getComputedStyle(c).backgroundColor]; for(var i = 0; i < q.length; i += 3) { q[i].childNodes[0].style.border = '1px solid ' + s[0]; q[i].childNodes[0].childNodes[0].style.backgroundColor = s[1]; q[i].childNodes[0].childNodes[1].childNodes[0].style.color = s[0]; } } function modifyPost(e) { if(e.parentNode.parentNode.childNodes[1].childNodes[1].textContent == '\n') return; if(GM_getValue(features[1][0], true)) e.innerHTML = e.innerHTML.replace(qFC, parseQFC); if(GM_getValue(features[2][0], true)) e.innerHTML = e.innerHTML.replace(yTV, parseYTV); if(GM_getValue(features[3][0], true)) { while(quote.test(e.innerHTML)) e.innerHTML = e.innerHTML.replace(quote, parseQuote); styleQuote(e); } if(GM_getValue(features[6][0], false) && GM_getValue(features[2][0], false)) convertYTV(e); var maxed = (document.getElementsByTagName('acronym').length > 0); if(maxed) return; if(GM_getValue(features[4][0], true) && GM_getValue(features[5][0], true)) quickQuote(e.parentNode.parentNode.getElementsByClassName('msgcommands')[0]); } function wipePost(e) { var yT = e.getElementsByTagName('youtube'); var quote = e.getElementsByTagName('quote'); for(var i = 0; i < quote.length; i ++) { var user = quote[i].getAttribute('user'); if(user != '') user = '-' + user; quote[i].innerHTML = '[q' + user + ']' + quote[i].getElementsByTagName('msg')[0].innerHTML + '[/q]'; } for(var i = 0; i < yT.length; i ++) yT[i].innerHTML = yT[i].getAttribute("video"); e.innerHTML = e.innerHTML.replace(/<\/?(quote|youtube|msg)(\s[^>]+)?>/g, ''); return e; } function parsePost() { var posts = document.getElementsByClassName('msgcontents'); if(posts == null) return; for(var i = 0; i < posts.length; i ++) { modifyPost(posts[i]); } } // Parse Thread function qFCLink() { uid = document.getElementById('uid'); uid.innerHTML = uid.innerHTML.replace(qFC, parseQFC); uid.childNodes[1].href = uid.childNodes[1].href.replace(/\/m=forum\/.+?\//, '/m=forum/'); } function quickReply() { var reg = /(?:.+?(?:(?=,)|$)){4}/; var func = function() { if(this.value.length > 2000) this.value = this.value.substring(0, 2000); }; var e = [document.createElement('div'), document.createElement('div'), document.createElement('div'), document.createElement('form'), document.createElement('textarea'), document.createElement('input')]; e[0].className = 'brown_box'; e[0].style.marginBottom = '5px'; e[1].className = 'subsectionHeader'; e[1].style.fontSize = '12px'; e[1].textContent = 'Quick Reply'; e[2].className = 'inner_brown_box'; e[3].method = 'post'; e[3].action = reg.exec(clip) + ',add'; e[3].style.textAlign = 'right'; e[4].name = 'contents'; e[4].style.display = 'block'; e[4].style.marginLeft = 'auto'; e[4].style.marginRight = 'auto'; e[4].style.height = '125px'; e[4].style.width = '675px'; e[4].id = 'quickReplyBox'; e[4].onkeyup = func; e[4].onblur = func; e[5].type = 'submit'; e[5].name = 'add'; e[5].value = 'Reply to thread'; e[5].style.height = '25px'; e[5].className = 'buttonmedium'; e.push(e[5].cloneNode(true)); e[6].name = 'preview'; e[6].value = 'Full post mode'; e[6].style.marginRight = '9px'; e[0].appendChild(e[1]); e[3].appendChild(e[4]); e[3].appendChild(e[5]); e[3].appendChild(e[6]); e[2].appendChild(e[3]); e[0].appendChild(e[2]); var msgs = document.getElementById('contentmsg'); msgs.parentNode.insertBefore(e[0], msgs.nextSibling); } function quickQuote(element) { if(new RegExp(/<a href=".+?">Quote Post<\/a>/).test(element.innerHTML)) return; var e = [document.createElement('br'), document.createElement('a')]; e[1].href = clip; e[1].textContent = 'Quote Post'; e[1].onclick = function() { var qRB = document.getElementById('quickReplyBox'); var name = this.parentNode.parentNode.childNodes[1].getElementsByTagName('a')[0].textContent; var text = this.parentNode.parentNode.nextSibling.nextSibling.childNodes[3].textContent; text = '[q-' + name + ']' + text.replace(/^\n+|\n+$/g, '') + '[/q]'; if(qRB.value != '') qRB.value += '\r\r'; qRB.value += text + '\r'; return false; }; element.appendChild(e[0]); element.appendChild(e[1]); } function edit(text, action, e) { e[1].innerHTML = e[1].innerHTML.replace(/\n$/, '<br>Editing post... '); var root = e[0].parentNode.parentNode.parentNode.parentNode; var orig = root.className; root.className = 'message moved'; modifyPost(e[0]); GM_xmlhttpRequest({ method: 'POST', url: action, data: 'contents=' + encodeURI(text[0]) + '&edit=true', headers: { "Content-Type": "application/x-www-form-urlencoded" }, onload: function(response) { var r = response.responseText; r = r.replace(/(\r\n|\n|\r)/gm, ''); r = r.replace(/.+?<table class="message msghighlight".+?"msgtime">/, ''); var timeStamp = r.replace(/<\/div>.+/, ''); timeStamp = timeStamp.replace('<br/>', ' <br/>') + '\n'; r = r.replace(/.+?<div class="msgcontents">/, ''); r = r.replace(/<\/div>.+/, ''); e[1].innerHTML = timeStamp; e[0].innerHTML = r; root.className = orig; modifyPost(e[0]); } }); return false; } function quickEditPost() { var box = this.parentNode.parentNode.nextSibling.nextSibling; if(box.getElementsByTagName('form')[0] != null) return; var timeStamp = box.childNodes[1]; if(new RegExp('Editing post...').test(timeStamp.innerHTML)) return; var element = box.childNodes[3]; var oldText = element.innerHTML; timeStamp.style.display = 'none'; var newText = wipePost(element).innerHTML.replace(/\n/g, ''); newText = newText.replace(/\s<br>/g, '\r'); var reg = [/(?:.+?(?:(?=,)|$)){4}/, /\d+,\d+$/]; var func = function() { if(this.value.length > 2000) this.value = this.value.substring(0, 2000); }; var e = [document.createElement('form'), document.createElement('textarea'), document.createElement('input')]; e[0].method = 'post'; e[0].action = reg[0].exec(clip) + ',edit,' + reg[1].exec(this.href); e[0].style.textAlign = 'right'; e[0].style.marginLeft = 'auto'; e[0].style.marginRight = 'auto'; e[0].style.width = '530px'; e[1].name = 'contents'; e[1].style.display = 'block'; e[1].style.marginLeft = 'auto'; e[1].style.marginRight = 'auto'; e[1].style.height = '200px'; e[1].style.width = '500px'; e[1].onkeyup = func; e[1].onblur = func; e[1].value = newText; e[2].type = 'submit'; e[2].name = 'edit'; e[2].onclick = function() { if(e[1].value == '') { return false; } element.removeChild(e[0]); element.innerHTML += e[1].value.replace(/\n/g, ' <br>'); timeStamp.style.display = ''; return edit([e[1].value, oldText], e[0].action, [element, timeStamp]); }; e[2].value = 'Edit post'; e[2].style.height = '25px'; e[2].style.marginBottom = '-10px'; e[2].className = 'buttonmedium'; e.push(e[2].cloneNode(true)); e[3].name = 'preview'; e[3].value = 'Full edit mode'; e.push(e[3].cloneNode(true)); e[4].name = 'cancel'; e[4].value = 'Cancel'; e[4].onclick = function() { element.removeChild(e[0]); element.innerHTML += oldText; timeStamp.style.display = ''; return false; }; e[4].style.marginRight = '12px'; e[0].appendChild(e[1]); e[0].appendChild(e[2]); e[0].appendChild(e[3]); e[0].appendChild(e[4]); element.textContent = ''; element.appendChild(e[0]); return false; } function quickEdit() { var msgCmds = document.getElementsByClassName('msgcommands'); for(var i = 0; i < msgCmds.length; i ++) { var e = msgCmds[i].getElementsByTagName('a')[0]; if(e == null) continue; if(e.textContent == 'Edit') e.onclick = quickEditPost; } } function parseThread() { var locked = new RegExp(/.+Locked.+/).test(document.getElementById('infopane').childNodes[3].innerHTML); var maxed = (document.getElementsByTagName('acronym').length > 0); if(GM_getValue(features[1][0], true)) qFCLink(); if(login || locked) return; if(new RegExp(/<a href=".+?edit.+?">Edit<\/a>/).test(document.getElementById('contentmsg').innerHTML) && GM_getValue(features[7][0], true)) quickEdit(); if(maxed) return; if(GM_getValue(features[4][0], true)) quickReply(); } // Parse Thread Highlight function parseThreadHighlight(thd) { var reg = /\d+,\d+$/; var threads = document.getElementsByClassName('thdnrml'); var thread = false; for(var i = 0; i < threads.length; i ++) { var threadLink = threads[i].getElementsByTagName('a')[0]; if(threadLink == null) continue; if(reg.exec(threadLink.href) == thd) { thread = threads[i]; break; } } if(!thread) return; var td = thread.getElementsByTagName('td'); var e = [document.createElement('div')]; e[0].style.border = '1px solid yellow'; e[0].style.borderWidth = '1px 0px 1px 1px'; e[0].style.height = '32px'; e[0].style.marginLeft = '-5px'; e[0].style.marginBottom = '-1px'; e[0].style.marginTop = '-1px'; e[0].style.padding = '3px 4px 0px 4px'; if(td[0].getElementsByTagName('img').length > 0) e[0].style.padding = '2px 4px 1px 4px'; // stupid picture messing up padding e.push(e[0].cloneNode(true)); e[1].style.borderWidth = '1px 0px 1px 0px'; e[1].style.height = '25px'; e[1].style.marginLeft = '-3px'; e[1].style.padding = '10px 0px 0px 3px'; e.push(e[1].cloneNode(true)); e[2].style.borderWidth = '1px 1px 1px 0px'; e[2].style.height = '30px'; e[2].style.marginRight = '-1px'; e[2].style.padding = '3px 0px 2px 3px'; for(var i = 0; i < td.length; i ++) { e[i].innerHTML = td[i].innerHTML; while(td[i].childNodes.length > 0) td[i].removeChild(td[i].firstChild); td[i].appendChild(e[i]); } } // Parse Profile function gm_search(t, e) { e.parentNode.parentNode.style.backgroundColor = '#2E2617' GM_xmlhttpRequest({ method: 'GET', url: e.href, onload: function(response) { e.parentNode.parentNode.style.backgroundColor = 'inherit'; var pR = response.responseText.replace(/(\r\n|\n|\r)/gm, ''); var reg = /(<div class="msgcontents">).+?(?=<\/div>)/; var pT = ''; while(reg.test(pR)) { pT += reg.exec(pR)[0].replace(/<[^<]+?>/g, '') + '\n'; pR = pR.replace(reg, ''); } if(t.test(pT)) e.parentNode.parentNode.style.backgroundColor = '#8a0a0a'; } }); } function search() { var t = document.getElementById('query').value; if(t == '') return false; t = new RegExp(t, 'i'); var e = document.getElementsByClassName('show_posts_by'); for(var i = 0; i < e.length; i ++) gm_search(t, e[i]); return false; } function searchProfile() { var history = document.getElementById('history'); if(history == null) return; var e = [document.createElement('form'), document.createTextNode('Search posts: '), document.createElement('input'), document.createElement('input')]; e[2].className = 'textinput'; e[0].style.marginTop = '16px'; e[0].style.marginBottom = '16px'; e[0].appendChild(e[1]); e[2].id = 'query'; e[0].appendChild(e[2]); e[3].type = 'submit'; e[3].className = 'buttonNew finduser_button buttonmedium'; e[3].value = 'Find'; e[0].appendChild(e[3]); e[0].onsubmit = search; history.parentNode.insertBefore(e[0], history); } function parseProfile() { if(GM_getValue(features[9][0], true)) searchProfile(); } // RAF Control Panel function rSAFLink() { var fLink = document.getElementById('community').parentNode; fLink = fLink.getElementsByTagName('ul')[0].childNodes[1]; rSAFL = fLink.cloneNode(true); rSAFL.childNodes[0].childNodes[0].textContent = 'RS Advanced Forums'; rSAFL.childNodes[0].href = 'forums.ws?rsaf'; fLink.parentNode.insertBefore(rSAFL, fLink.nextSibling); } function rSAFCP() { document.getElementById('article').childNodes[1].childNodes[1].childNodes[1].childNodes[1].textContent = 'RuneScape Advanced Forums Script'; var location = document.getElementsByClassName('location'); for(var i = 0; i < location.length; i ++) location[i].childNodes[5].childNodes[0].textContent = 'RuneScape Advanced Forums Script'; var infopane = document.getElementById('infopane') while(infopane.childNodes[3].childNodes[1].childNodes.length > 3) infopane.childNodes[3].childNodes[1].removeChild(infopane.childNodes[3].childNodes[1].firstChild); infopane.childNodes[3].childNodes[1].removeChild(infopane.childNodes[3].childNodes[1].childNodes[1]); infopane.childNodes[3].style.marginTop = '0px'; infopane.childNodes[3].childNodes[1].childNodes[1].textContent = 'Release #' + rN[0] + ': ' + rN[1]; infopane.childNodes[1].textContent = 'RuneScape Advanced Forums'; infopane.parentNode.removeChild(document.getElementById('contentfrm')); var e = [document.createElement('div'), document.createElement('div'), document.createElement('div')]; e[0].className = 'brown_box'; e[0].style.marginBottom = '5px'; e[1].className = 'subsectionHeader'; e[1].style.fontSize = '12px'; e[1].textContent = 'Welcome'; e[2].className = 'inner_brown_box'; e[2].textContent = 'You are running RuneScape Advanced Forums: Release #' + rN[0] + ' (' + rN[1] + '). This script has been inactive for ~ 4 years but is now being rewritten. Previous features are expected in the next version. Here you can enable or disable certain features of the script.'; e[0].appendChild(e[1]); e[0].appendChild(e[2]); e.push(e[0].cloneNode(true)); e[3].childNodes[0].textContent = 'Features'; e[3].childNodes[1].textContent = 'Click to toggle a feature.'; e[3].childNodes[1].appendChild(document.createElement('br')); e[3].childNodes[1].appendChild(document.createElement('br')); e[3].childNodes[1].appendChild(document.createElement('hr')); var i = 0; var feature = e[3].childNodes[1]; feature.appendChild(document.createElement('table')); feature.lastChild.appendChild(document.createElement('tbody')); feature = feature.lastChild.lastChild; while(i < features.length) { var enabled = GM_getValue(features[i][0], features[i][2]); feature.appendChild(document.createElement('tr')); var tr = feature.lastChild; tr.style.height = '50px'; tr.appendChild(document.createElement('td')); tr.lastChild.style.width = '10%'; tr.lastChild.appendChild(document.createElement('a')); tr.lastChild.lastChild.appendChild(document.createTextNode(enabled.toString().toUpperCase())); tr.lastChild.lastChild.style.textDecoration = 'none'; if(!enabled) tr.lastChild.lastChild.style.color = 'red'; if(enabled) tr.lastChild.lastChild.style.color = 'green'; tr.lastChild.lastChild.href = window.location.href; tr.lastChild.lastChild.id = 'feature_' + i; tr.lastChild.lastChild.onclick = function() { var i = parseInt(this.id.substring(8)); var enabled = GM_getValue(features[i][0], features[i][2]); if(enabled != features[i][2]) GM_deleteValue(features[i][0]); else GM_setValue(features[i][0], !enabled); enabled = GM_getValue(features[i][0], features[i][2]); if(enabled) this.style.color = 'green'; else this.style.color = 'red'; this.textContent = enabled.toString().toUpperCase(); return false; }; tr.appendChild(document.createElement('td')); tr.lastChild.style.width = '20%'; tr.lastChild.appendChild(document.createTextNode(features[i][0])); tr.appendChild(document.createElement('td')); tr.lastChild.style.width = '80%'; tr.lastChild.style.textAlign = 'right'; var text = features[i][1].split("."); for(var t = 0; t < text.length; t ++) { if(text[t] == '') break; if(t > 0) tr.lastChild.appendChild(document.createElement('br')); tr.lastChild.appendChild(document.createTextNode(text[t] + '.')); } i ++; } e.push(e[0].cloneNode(true)); e[4].childNodes[0].textContent = 'A Note to Google Chrome Users'; e[4].childNodes[1].textContent = 'Google Chrome does not fully implement the features of Greasemonkey. The values stored here are maintained within the Greasemonkey extension in Firefox, whereas Chrome does not have this feature. To compensate, Google Chrome users will store these variables in HTML5\'s localStorage. This means that if you uninstall this script while using Google Chrome, the variables will remain. By default, no variables are stored unless a setting is toggled. To fully clear the variables in order to do a clean uninstall, press the link below. This will also purge the variables from other browsers.'; e[4].lastChild.appendChild(document.createElement('br')); e[4].lastChild.appendChild(document.createElement('br')); e[4].lastChild.appendChild(document.createElement('a')); e[4].lastChild.lastChild.href = window.location.href; e[4].lastChild.lastChild.textContent = 'Purge variables'; e[4].lastChild.lastChild.onclick = function() { window.localStorage.clear(); for(var i = 0; i < features.length; i++) { var link = document.getElementById('feature_' + i); var enabled = GM_getValue(features[i][0], features[i][2]); if(enabled) link.style.color = 'green'; else link.style.color = 'red'; link.textContent = enabled.toString().toUpperCase(); } return false; }; infopane.parentNode.appendChild(e[0]); infopane.parentNode.appendChild(e[3]); infopane.parentNode.appendChild(e[4]); } // Determine Components function determineComponent() { rSAFLink(); var rSAF = /forums\.ws\?rsaf/; var postView = /forums\.ws\?(\d{1,3},){3}\d+(,.+|$)/; var threadView = /forums\.ws\?(\d{1,3},){3}\d+(,(?!add|edit).+|$)/; var threadHighlight = /thd,(.+)/; var profileView = /users.ws/; if(login && GM_getValue(features[0][0], true)) quickLogIn(); if(rSAF.test(clip)) return rSAFCP(); if(postView.test(clip)) parsePost(); if(threadView.test(clip)) parseThread(); if(threadHighlight.test(clip) && GM_getValue(features[8][0], true)) parseThreadHighlight(threadHighlight.exec(clip)[1]); if(profileView.test(clip)) parseProfile(); } determineComponent();
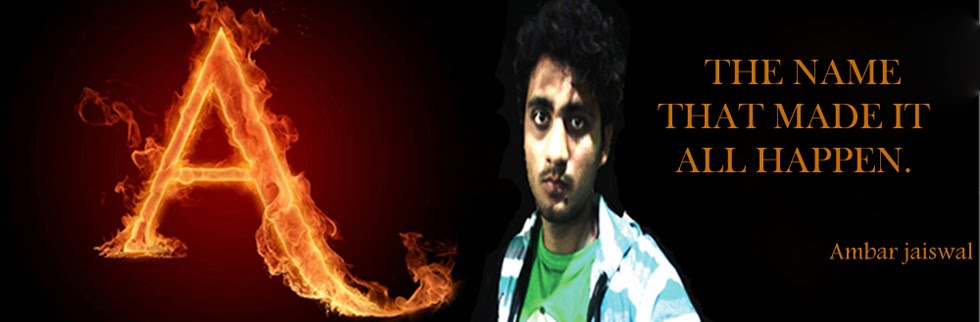
Mozilla add on,User script,Grease Monkey Script, greasemonkey userscripts, updater userscripts mafia wars userscripts mafia wars autoplayer userscripts mafia wars wall userscripts scripts userscripts travian greasemonkey greasemonkey download greasemonkey facebook greasemonkey tutorial greasemonkey youtube greasemonkey travian greasemonkey chrome greasemonkey mafia wars greasemonkey mafia wars autoplayer
Thursday, September 22, 2011
RS Advanced Forums
Subscribe to:
Post Comments (Atom)
0 comments:
Post a Comment